Powershell is all about quick and concise commands for achieving desired system administration tasks. Finding CPU utilization of local and remote computers is one such task. We can easily find the CPU usage of a computer using a mix of Powershell commands and scripts.
In this document, we will list the following:
- Powershell command to find CPU utilization of local computer using CimInstance
- Powershell command to find CPU utilization of local computer using WmiObject
- Powershell script that finds CPU usage and memory usage in a continuous loop
With these Powershell commands, you can pull in CPU util quickly. This can be done for local computers and remote computers. For remote computers, we do suggest using Powershell to use a PS shell on the remote computer.
Find CPU Utilization using CimInstance
The simplest approach to find average CPU utilization of a local computer is by typing the following Powershell command:
Get-CimInstance win32_processor | Measure-Object -Property LoadPercentage -Average
The result of this command with Maximum and Minimum filters looks like the screen capture displayed below.

The command output displays the count of processors in the computer and the average CPU utilization of these processors on the computer.
Find CPU Utilization using WmiObject
WmiObject can be used to fetch CPU utilization on a real time basis.
If you wish to get the average CPU utilization only, you can qualify the Wmi Object command by adding a filter as per the command below.
Get-WmiObject Win32_Processor | Measure-Object -Property LoadPercentage -Average | Select Average
This WmiObject command will provide average CPU utilization on the computer as per the command output displayed below.

Get-WmiObject Win32_Processor | Measure-Object -Property LoadPercentage -Average | Select Maximum
This Wmi Object command has a filter for ‘Maximum’ CPU utilization. It will display the Maximum CPU utilization on the computer.
Get-WmiObject Win32_Processor | Measure-Object -Property LoadPercentage -Average | Select Minimum
The minimum filter on the WmiObject command will provide minimum CPU utilization recorded on a real time basis.
The result of this command with Maximum and Minimum filters looks like the screen capture displayed below.

CPU utilization on continuous loop
The Powershell script below will display the CPU and memory utilization of a remote or local computer on a continuous basis.
while($true) {
$date = Get-Date -Format “yyyy-MM-dd HH:mm:ss”
$cpuTime = (Get-Counter ‘\Processor(_Total)\% Processor Time’).CounterSamples.CookedValue
$availMem = (Get-Counter ‘\Memory\Available MBytes’).CounterSamples.CookedValue
$date + ‘ > CPU: ‘ + $cpuTime.ToString(“#,0.000”) + ‘%, Avail. Mem.: ‘ + $availMem.ToString(“N0”) + ‘MB (‘ + (104857600 * $availMem / $totalRam).ToString(“#,0.0”) + ‘%)’
Start-Sleep -s 10
}
The script above can be pasted on Powershell command prompt also. When the command executes, CPU and Memory utilization will be recorded and shared after intervals of 10 seconds. Note the Start-Sleep variable timer. It is set to 10 seconds in this command. You can alter the Start-Sleep parameter to increase or decrease the interval at which CPU and memory usage is recorded and shared.
The command output is shared below in the screen capture taken from the Powershell command windows.
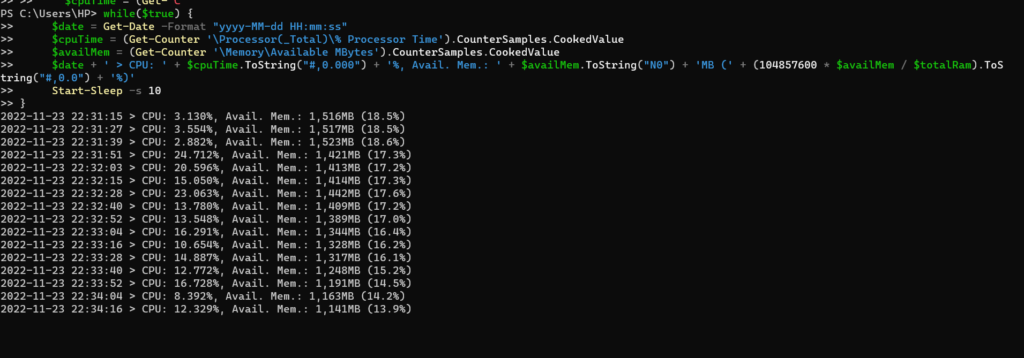
You can break this CPU and memory tracker by pressing Ctrl C.
To monitor CPU and memory for a remote computer, we suggest opening a PS Session on the remote computer. Once a Powershell remote connection is created, you can run any of the commands shared above.
Suggested Powershell Tutorials
You may like to read more content related to Powershell below:
- How to get the last line of a file using Powershell?
- How to get event logs for an event using Powershell?
- How to export event logs to CSV file using Powershell?
- How to find the properties of an event log in Powershell?
- How to get the first few lines of a file using Powershell?
- How do I check if a directory exists in Powershell?
- How to find running services in Powershell?
- How to enable Remote Desktop using Powershell?
- Get CPU temperature using Powershell
- Get all hotfixes installed by date in Powershell
- How to find stopped services using Powershell?
Rajesh Dhawan is a technology professional who loves to write about Cyber-security events and stories, Cloud computing and Microsoft technologies. He loves to break complex problems into manageable chunks of meaningful information.