Powershell can be used to download files from a URL on the web. You can download the files to specific folder locations on the computer.
Introduction
Powershell 3 offers simpler and straightforward ways to download files from the web to a specific location on the computer.
For our example, we will download the Powershell MSI installer file from the GitHub URL and place it under a folder D:\PS. We will use the Invoke-webrequest approach and the Invoke-RestMethod approach to download the external file.
For this example, we will perform the following tasks:
- We will pick the URL of the Powershell MSI installer from the GitHub page. This URL will be assigned to a variable $source.
- We will create a folder on our computer to which the MSI installer of Powershell will be copied. Let us name it the PS folder under D:. So, the target for this operation becomes D:\PS folder. This folder location will be used to copy the MSI file to PS1.msi file. So, our target becomes D:\PS\PS1.msi. This target is assigned to a variable $target.
- We will use the Invoke-webrequest method to copy from file from source to target.
- We will validate the successful placement of the file by checking the content of the folder.
We will repeat the same process for the Invoke-RestMethod approach. For this, we will use the source as $source1 and the target as $target1. The file to copy will be the MSI file for Powershell 7.3.1. The file will be copied to the target file D:\PS\PS1.msi.
We will perform both tasks below. You can replicate these on your system for a quick run.
Using Invoke-webrequest to copy file from a URL to a file on local computer
The full list of commands that you need to use on the Powershell command prompt is shared below.
$source=”https://github.com/PowerShell/PowerShell/releases/download/v7.3.1/PowerShell-7.3.1-win-x64.msi”
$target=”d:\ps\powershell.msi”
Invoke-WebRequest -Uri $source -OutFile $target -UseBasicParsing
In the commands above, we have created two variables. $source is used to store the URL from which we want to download the file. $target is the variable that stores the file that would be copied with its full name. Once the two variables have been defined, we will use the Invoke-webrequest to download the file from the source to the target.
When you execute these commands, you would see the file being copied on a real-time basis. The screenshot below captures the screen while the file is being downloaded. The yellow progress bar indicates the number of bytes that have been downloaded.

Once the file has been downloaded, the progress bar will disappear. If you run into any errors, you will see the error message on the console in red.
At this point, we will validate the content of the folder in which we downloaded the file. The command to check the contents of a folder is shared below:
Get-childitem d:\ps\
The output of the complete command set, including the Get-Childitem cmdlet is displayed below in the screenshot.

You can validate that the powershell.msi file of 106 MB size has been downloaded from the source link to the target folder.
Download file from a URL using Invoke-RestMethod in Powershell
Invoke-RestMethod can be used to download files from a URL on the web to a folder file location on the local computer.
For this operation, we will follow the process below:
- We will download the Powershell 7.3.1 MSI folder from the GitHub page
- The file will be copied under D:\PS and named PS1.msi
- We will validate the successful download of the file by checking the command output of Get-Childitem cmdlet.
The first set of commands that we need to place on the Powershell command prompt are shared below:
$source=”https://github.com/PowerShell/PowerShell/releases/download/v7.3.1/PowerShell-7.3.1-win-x64.msi”
$target=”d:\ps\ps1.msi”
Invoke-RestMethod -Uri $source -OutFile $target -UseBasicParsing
When the file download starts, you will see a progress bar on the console. The screenshot below captures the progress bar when we have given all three commands on the Powershell window.

To validate the download of the file, we will use the Get-Childitem cmdlet as displayed in the command below.
Get-childitem d:\ps\
The combined output of all these commands is displayed below in the screenshot.
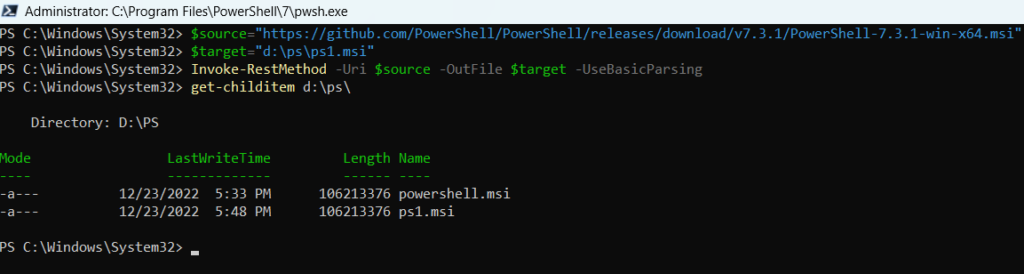
You can see that we were able to download PS1.msi file from the GitHub page of Powershell to a local folder on the computer. You could use the Invoke-RestMethod or Invoke-WebRequest to download any available file from the web to a local folder.
Download files that require authentication details using Powershell
There is a possibility that you may have to share authentication details before downloading files from a web server. This may be true for security updates for some companies.
We can still use the Invoke-Webrequest and Invoke-Restmethod methods to download the files. We will need to provide the authentication details on the command itself. For the sake of simplicity, you can place the credentials in a variable like this:
$creds=”techepages\root_user_89″
We can then place these credentials on the command line.
So, in case we have to share the authentication details for downloading files from a URL on the web, we will use any of these two commands below:
Invoke-WebRequest -Uri $source -OutFile $target -UseBasicParsing -credential $creds
Invoke-RestMethod -Uri $source -OutFile $target -UseBasicParsing -credential $creds
We will still need to define $source and $target before using Invoke-webrequest or Invoke-RestMethod commands to download the file from a URL to the local folder.
When you use the ‘credential’ parameter on the command line of Powershell, you will need to share the password on the Powershell window to download the file from the source to the target.
Summary
In this Powershell tutorial, we have seen that the files can be downloaded from a URL to the local folder using Invoke-webrequest or Invoke-RestMethod. We have also seen how we could download files from a server that may require credentials or authentication before allowing te files to download.
Suggested Powershell Tutorials
The following Powershell tutorials help in performing basic system administration tasks on Windows computers.
- How to find disk block size in Powershell?
- How to list installed printers in Powershell?
- How to find current directory path in Powershell?
- How do I convert epoch time to date in Powershell?
- How to find the public IP address using Powershell?
- How to find the boot directory using Powershell?
- How to find DoH DNS Servers using Powershell?
- How to get sid from the user account in Powershell?
- How to get system boot time in Powershell?
- How to append to a file in Powershell?
Rajesh Dhawan is a technology professional who loves to write about Cyber-security events and stories, Cloud computing and Microsoft technologies. He loves to break complex problems into manageable chunks of meaningful information.